Step 1 - Basic Structure#
Before we can design our game, we need to do a few things to set rubato up.
First, follow the setup guide, naming your file main.py
.
Then, download and extract these
files
into the same directory as your main.py
file (so you have main.py
and the files
folder in the same directory.)
At this point, your main.py
file should look like this:
1import rubato as rb
2
3# initialize a new game
4rb.init()
5
6# begin the game
7rb.begin()
Running main.py
using python main.py
should result in a window similar to this appearing:
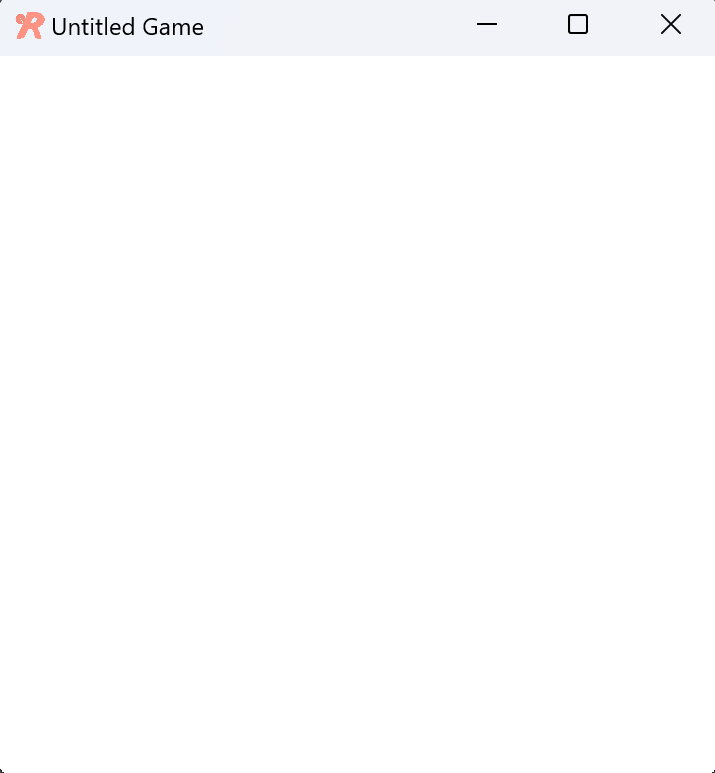
rb.init()
is the initializer function for the library.
It will ensure that rubato can communicate with the computer hardware and
set up a window for you.
rb.begin()
actually runs the game loop. The loop will
handle all of the rendering, player logic, etc. Without calling it, nothing happens.
- To customize your game window, you can pass in a few parameters. For now, let's:
Give our window a name
Change its resolution
Make it fullscreen
Replace the previous rb.init()
call with this:
3# initialize a new game
4rb.init(
5 name="Platformer Demo", # Set a name
6 res=(1920, 1080), # Set the window resolution (in pixels).
7 fullscreen=True, # Set the window to be fullscreen
8)
At this point, running the game should look like this (full screen and white). To quit the game either quit like any
other program or press Ctrl+C
in the terminal.
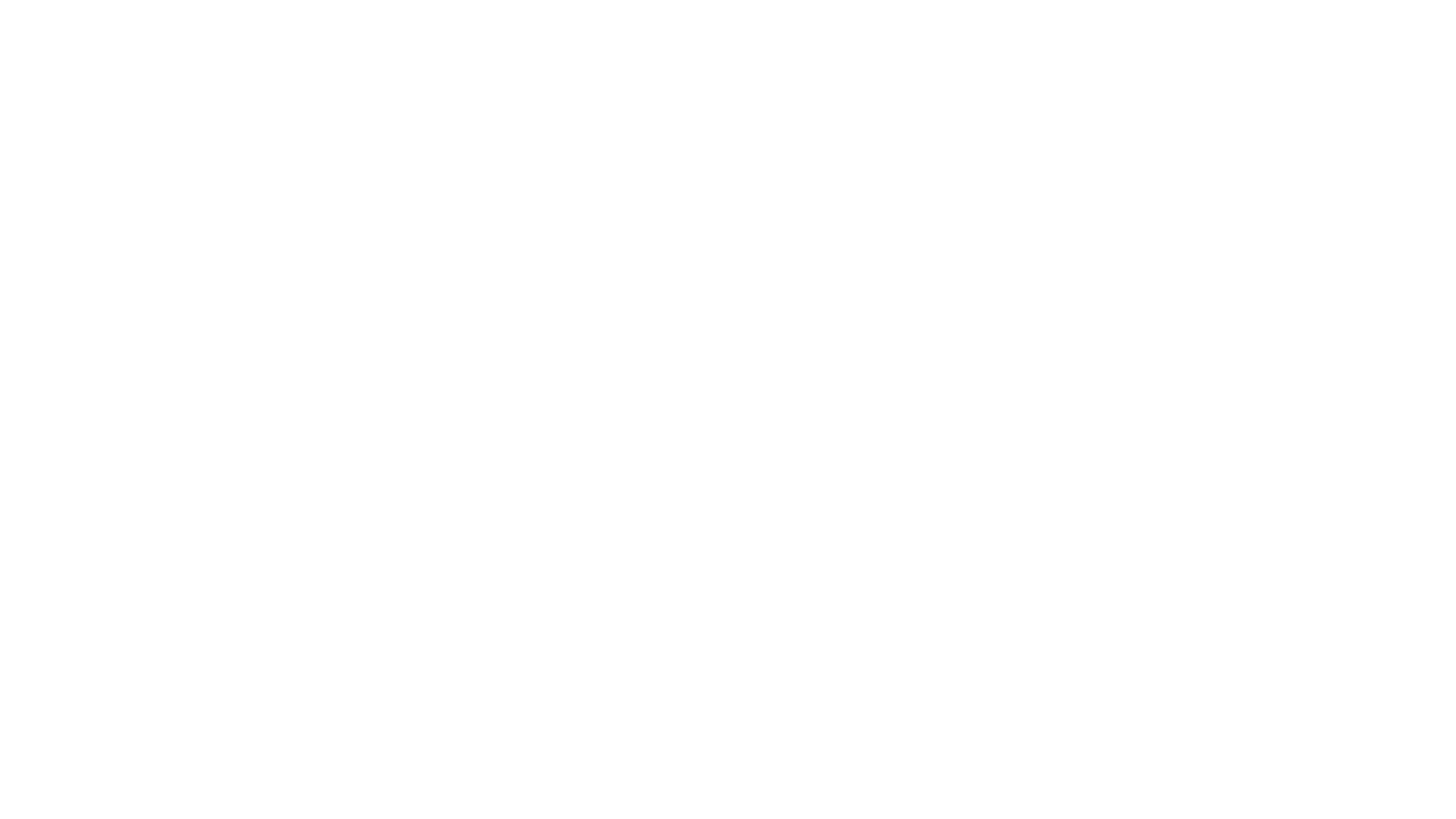
Here is what your main.py should look like:
1import rubato as rb
2
3# initialize a new game
4rb.init(
5 name="Platformer Demo", # Set a name
6 res=(1920, 1080), # Set the window resolution (in pixels).
7 fullscreen=True, # Set the window to be fullscreen
8)
9
10# begin the game
11rb.begin()
If you made it here, great! We're ready to build the platformer. Next, we'll create a player and add him to the game.